Bitcoin Wallet Service is a free open source service that allows merchants to send bitcoin and retain custody of their funds.
Resources
Source Code![]() | https://github.com/blockonomics/wallet_service |
Youtube Video![]() | https://youtu.be/GpN6B7ca5-I |
Requirements
- Linux System
- Python 3.8
- Git
- Postman ( For Testing )
Initial Setup
The code repository of the Self Hosted Wallet Service is available on Github here. Step-by-step instructions regarding how to install and configure the wallet service are provided in the README of the repository; if you want to test the wallet service, set use_testnet to True, otherwise set it to False for mainnet.
Creating Wallet
For creating a wallet we offer a CLI call python wallet_service_cli.py createwallet <wallet_password>, where <wallet_password> indicates the newly-created password for wallet authorization. The wallet details including the wallet ID, password, seed of the wallet (To recover your bitcoin wallet ), and Xpub Key will be returned as a response following the command execution.
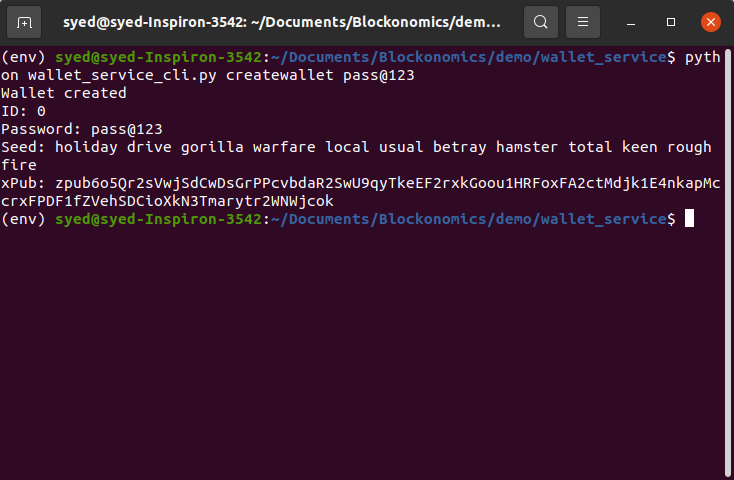
Integrating with your Store
After creating the wallet, you can integrate the wallet with your store. The stores can be created or updated under Blockonomics Merchants > Stores. To create a store, you will need the Xpub key you obtained when you created your wallet, and, then, you will need a sample receiving address. For generating a receiving address, we can use the Python CLI command python wallet_service_cli getunusedaddress <wallet_id> <wallet_password>
. Once the service has been integrated with your store, you can start receiving bitcoins through the new API address and http callbacks.
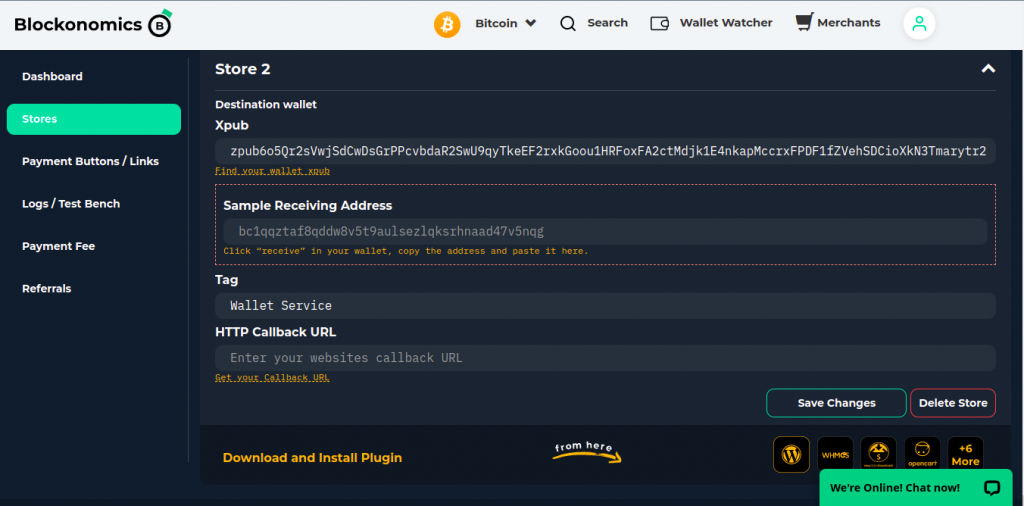
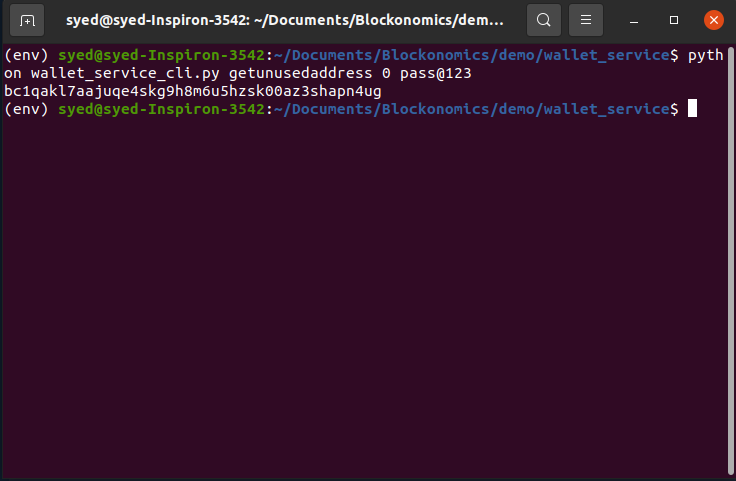
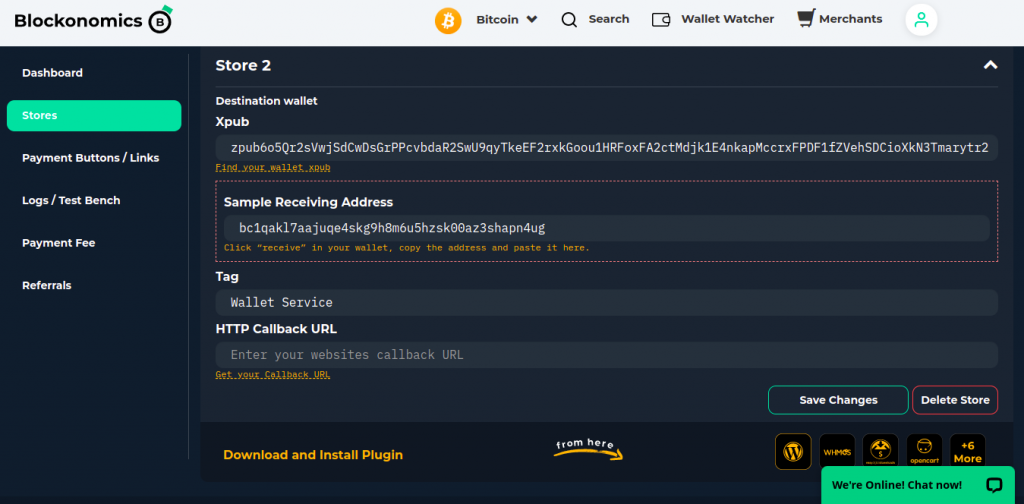
Start your Service
Once you’ve completed the necessary configurations and installations, launch your wallet service using python wallet_service_api.py
. The wallet service will run on localhost, listening on port 8080 by default. In the server, you can see logs being updated after every API call and you can now use APIs for sending bitcoins through Wallet Service.
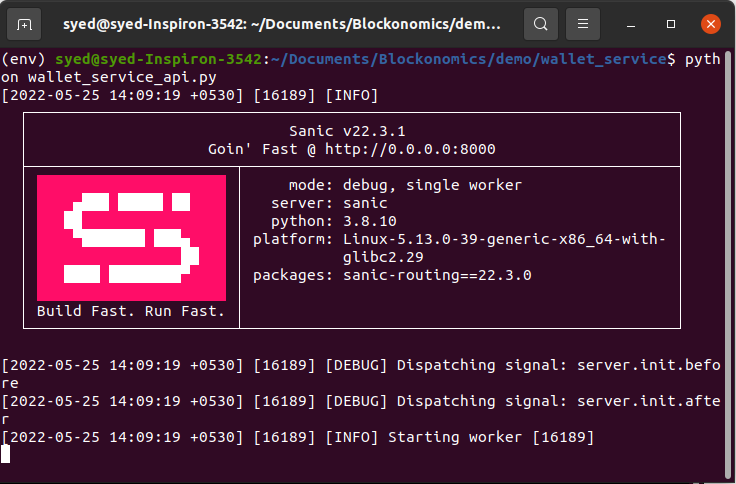
Working
In wallet service, the api/presend can be used to estimate the fee for your transaction, which you can then analyze, and schedule your transaction in the queue with the help of the api/send. Here is how internally the send process works:
- All incoming sends are added to a queue
- The service attempts to batch send the queue every 5 minutes (send frequency)
- If the fa_ratio (fee to amount ratio) during send attempt is less than 5% (fa_ratio_limit), then the send is triggered else the send requests keep waiting in the queue
- During the next send attempt (after 5 more minutes), fa_ratio_limit doubles to 10%
The above process continues till the queue is cleared or fa_ratio_max (50%) is reached. There are two principles behind the working of wallet service. First, sends are queued and batched so that your customers don’t pay very high fee for sends. Second, the service also avoids high waiting time for sends by increasing fee as time passes.
Test your Wallet Service
Start testing your wallet by setting the use_testnet value to True using python wallet_service_cli.py setapiconfig use_testnet True
. After configuring the use_testnet, you must create a new wallet for the testnet bitcoins. The procedure is the same as for the mainnet. Bitcoin faucets like this one offer test bitcoins for you to add to your wallet. In order to test APIs provided by the wallet service, you can use a tool called Postman, which allows you to make API calls with all types of parameters.
Testing APIs
1. presend
The api/presend is a POST request API call is used to get an estimated fee for your transaction, with the help of this API you can analyze your transaction and transaction fee. This API requires 5 parameters in the body as JSON format during the post request call, the required parameters are
- addr (bitcoin receiving address)
- btc_amount
- wallet_id
- wallet_password
- api_password
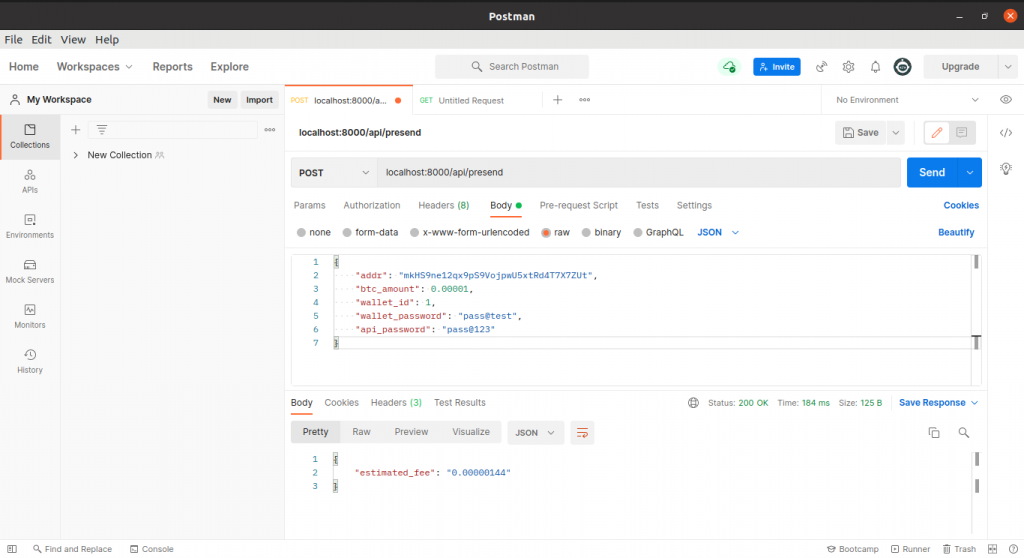
2. send
The api/send is also a POST request API call which schedules your transaction in the queue. This API call also requires the 5 parameters same as the presend API call.
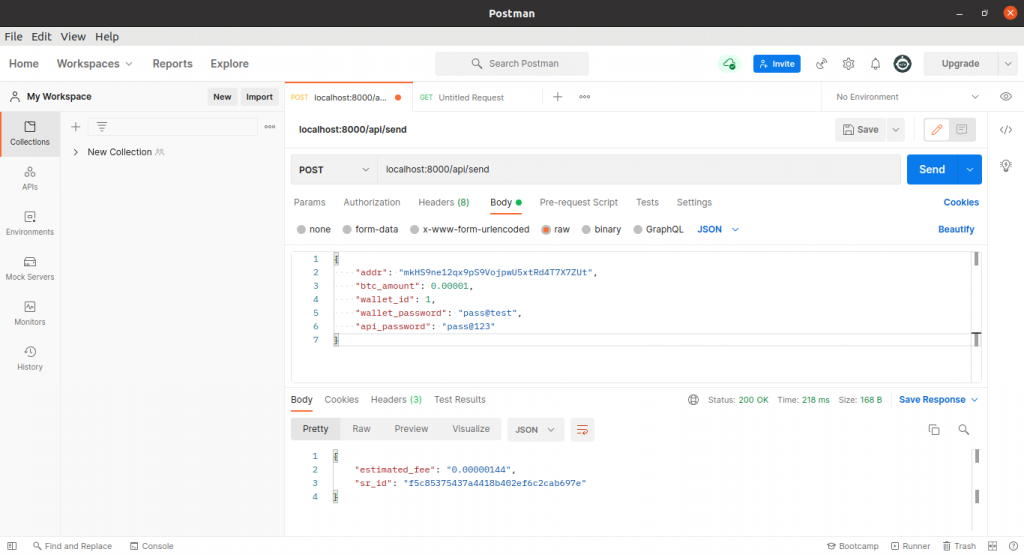
3. queue
Queue – api/queue is a GET request API call with which you can fetch the current queue of transactions. In response, we can get the queue of transaction ids, total BTC amount, total transaction fee, fa_ratio, fa_ratio_limit and the next send attempt timer.
Let us see a demo of working of the queue
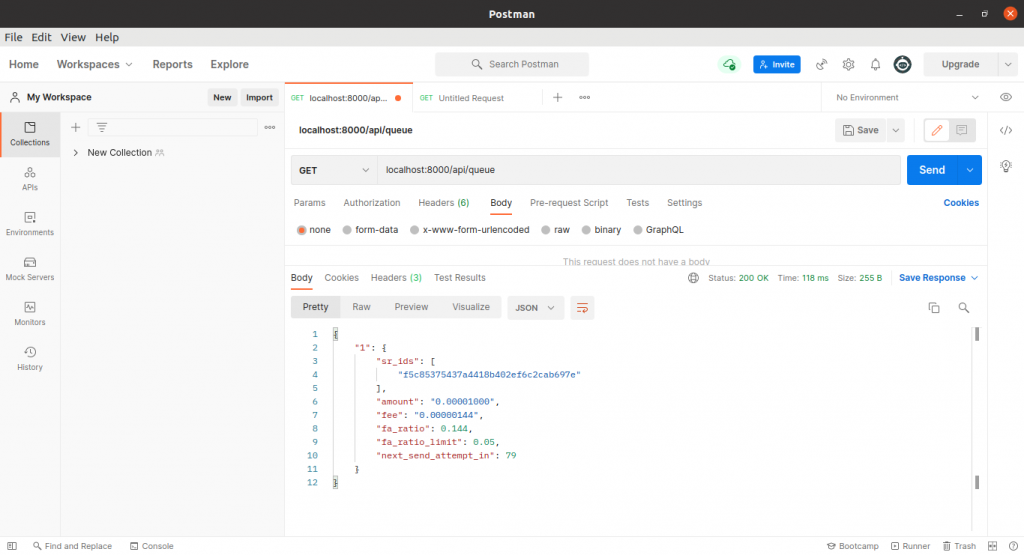
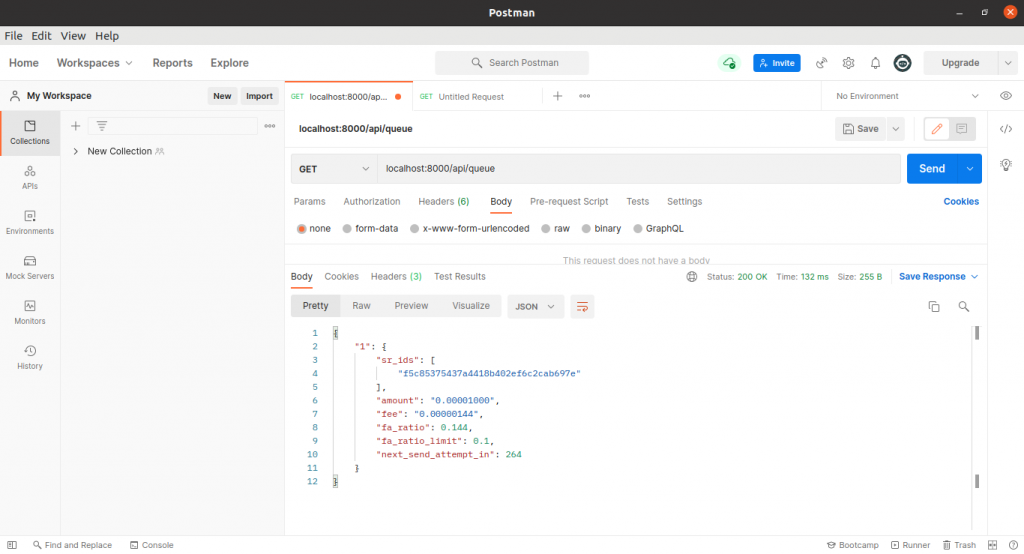
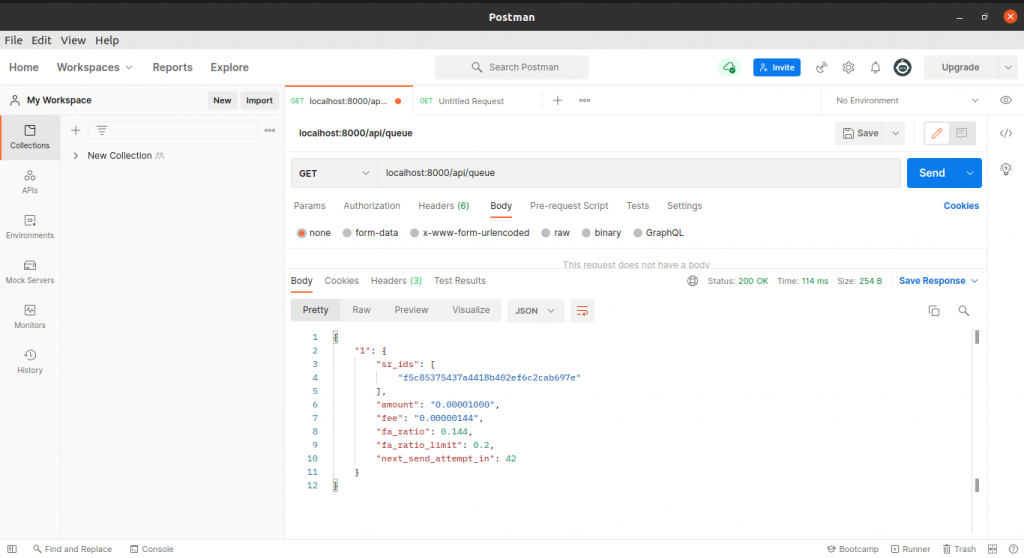
4. detail
To view the details of a transaction we can make use of the details GET request API call – api/detail/<sr_id>, which takes the transaction id (sr_id) as query parameter and returns the details of the transaction such as receiving address , bitcoin amount and the time stamp.
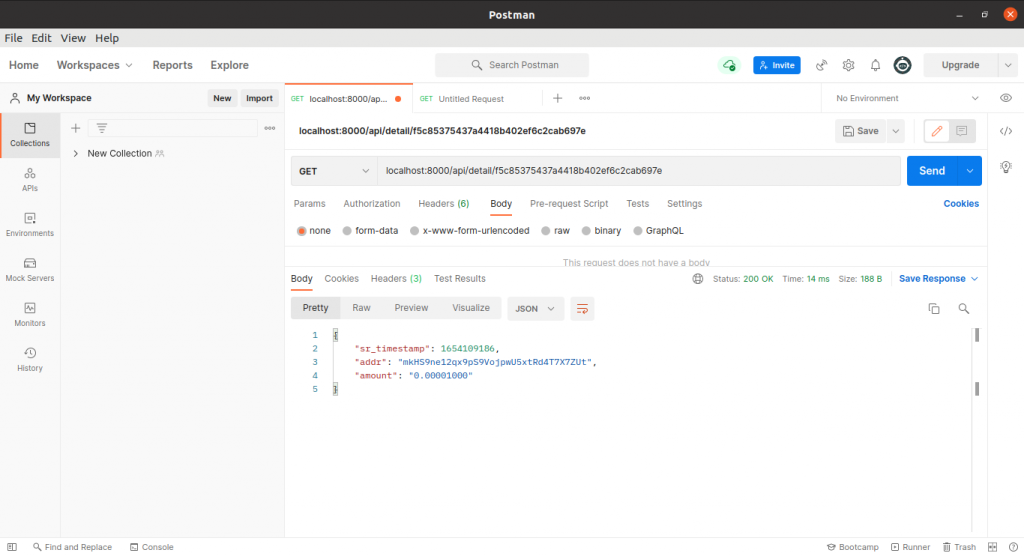
The End
We have successfully installed and configured the wallet service in our system, which will help us to send Bitcoin payments. We also learned about the logic behind fa_ratio and fa_ratio_limit, and also made API calls to test the wallet service with testnet.